📙 1. 문제
Link : https://school.programmers.co.kr/learn/courses/30/lessons/150370
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
문제 설명
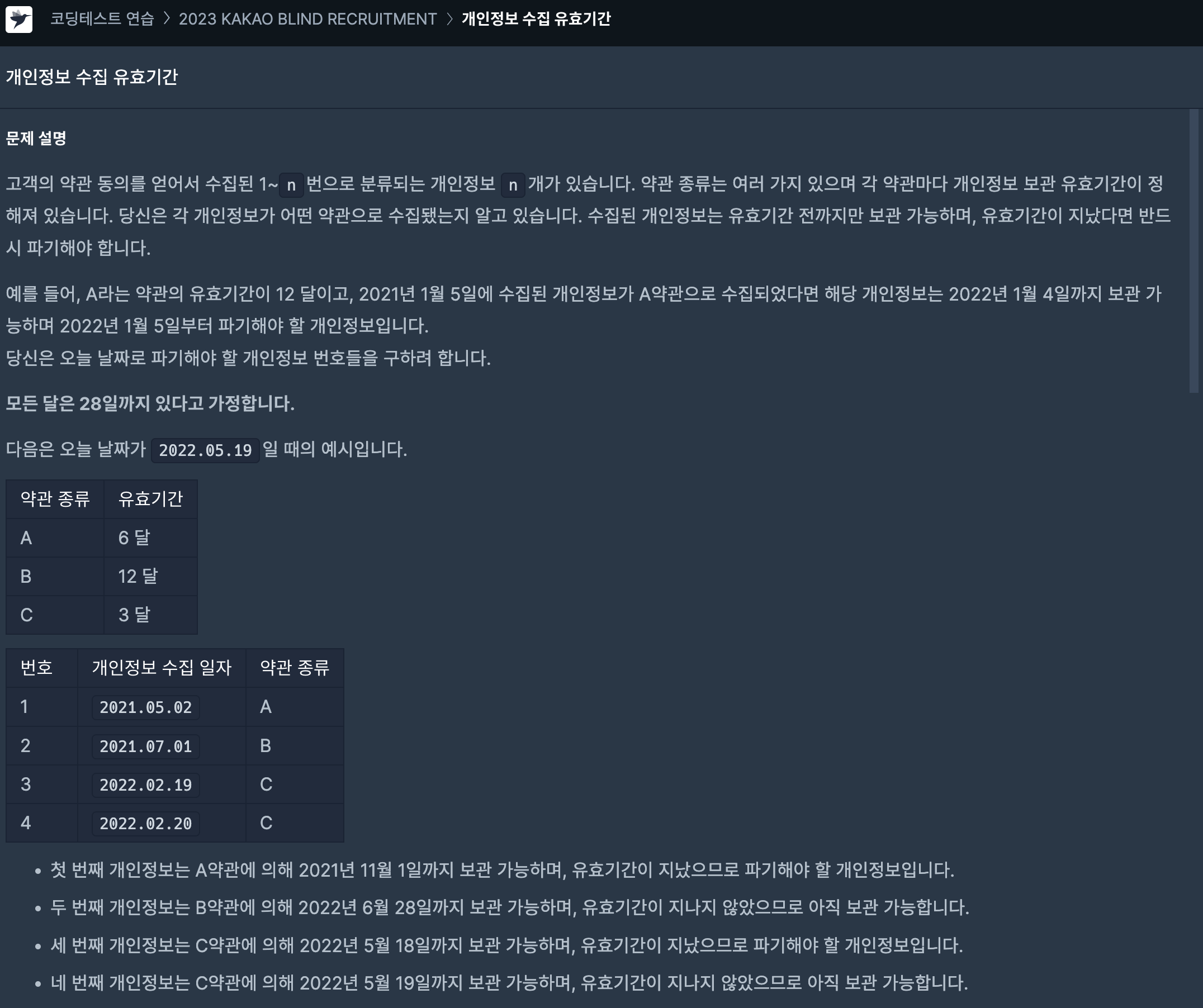
풀이 1 : 내 풀이
풀이 과정
1. 각 개인정보 별 유효기간을 구한다. (privacies와 terms의 일치하는 타입 이용)
2. 1에서 구한 유효기간 날짜와 오늘 날짜를 비교한다. (오늘 날짜가 더 이후라면, answer배열에 push)
1번, 유효기간 구하는 함수 datePlus를 만들었다. 시작 날짜 startDate와 더할 기간 period를 구한다.
period만큼 월 수를 더하고, 여기서 예외처리가 중요하다. 12로 나눈 값만큼 연도에 더하고, 12로 나눈 나머지만큼의 값만 가진다.
그리고, 일자를 1씩 빼준다. 여기서, 2020년 1월 1일같은, 경계선에 대한 예외처리가 중요하다.
2번, 유효기간 날짜와 오늘 날짜를 비교하는 함수 compare함수를 만들었다. 오늘 날짜가 더 이후라면 expired를 반환하고, 아니라면 notExpired를 반환한다.
const datePlus = (startDate,period) => {
// 월 +1
let [year,month,date] = startDate.split('.').map(v=>+v);
month+= +period;
year += (parseInt(month/12));
month = month % 12;
date --;
if(date===0){
date=28;
month--;
}
if(month===0){
year--;
month=12;
}
return {year,month,date};
}
const compareDate = (expiration,today) => {
if(today.year>expiration.year) return 'expired';
if(today.year===expiration.year && today.month>expiration.month) return 'expired';
if(today.year===expiration.year && today.month===expiration.month && today.date>expiration.date) return 'expired';
return 'notExpired'
}
function solution(today, terms, privacies) {
var answer = [];
// 1. 유효기간 구하기
// 2. 유효기간 < today인지 구하기
const timesType={};
terms.forEach((term)=>{
term = term.split(' ')
timesType[term[0]] = term[1]
});
const [year,month,date] = today.split('.').map(v=>+v)
today = {year,month,date}
privacies = privacies.map((privacy,index)=>{
const [startDate,type] = privacy.split(' ');
const expiration = datePlus(startDate,timesType[type])
if (compareDate(expiration,today)==='expired'){
answer.push(index+1)
}
})
return answer;
}
🤔 2. 느낀 점
생각한대로 알고리즘을 짜고, 알고리즘대로 코드를 작성해내는 '구현' 문제이다. 그 알고리즘을 짜는 것이 상당히 중요하다는 것을 느꼈다. '유효기간을 구하고, 오늘 날짜와 비교하면 되겠지?' 생각에서 이를 단계로 만들어내는 능력. 그것이 알고리즘이구나.
그리고, 모든 테스트케이스를 통과하려면 각 조건들을 확인하여 예외처리를 잘 해야한다는 것을 느꼈다.
'코딩 테스트 > 프로그래머스' 카테고리의 다른 글
[프로그래머스] 수식 최대화 (0) | 2023.05.26 |
---|---|
[프로그래머스] 표 병합 (0) | 2023.05.21 |
[프로그래머스] 코딩테스트 공부 (0) | 2023.05.20 |
[프로그래머스] 표현 가능한 이진트리 (1) | 2023.05.19 |
[프로그래머스] 거리두기 확인하기 (0) | 2023.05.14 |
[프로그래머스] 방금 그곡 (1) | 2023.05.13 |
[프로그래머스] 후보키 (0) | 2023.05.12 |
[프로그래머스] 문자열 압축 (0) | 2023.05.11 |
📙 1. 문제
Link : https://school.programmers.co.kr/learn/courses/30/lessons/150370
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
문제 설명
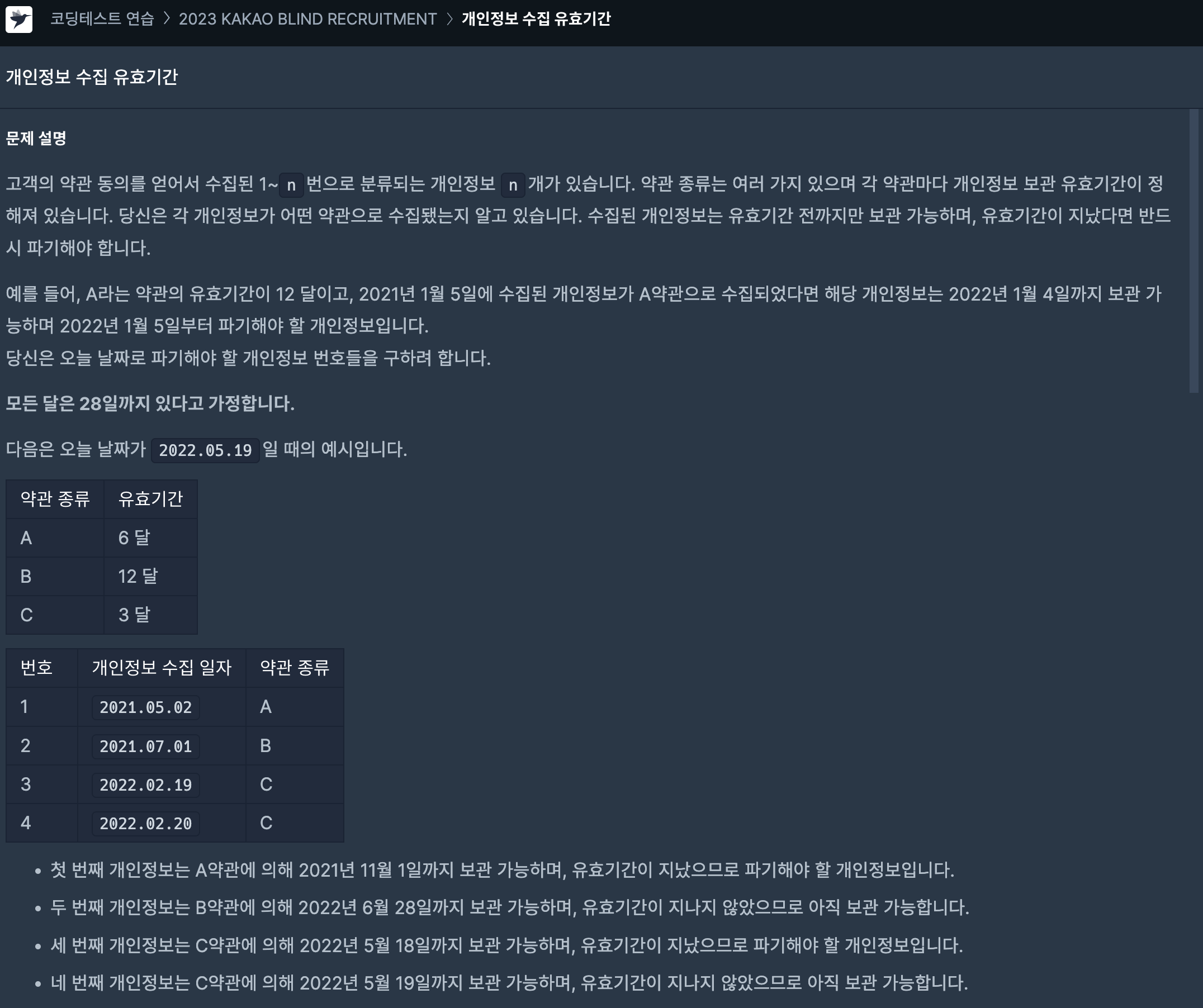
풀이 1 : 내 풀이
풀이 과정
1. 각 개인정보 별 유효기간을 구한다. (privacies와 terms의 일치하는 타입 이용)
2. 1에서 구한 유효기간 날짜와 오늘 날짜를 비교한다. (오늘 날짜가 더 이후라면, answer배열에 push)
1번, 유효기간 구하는 함수 datePlus를 만들었다. 시작 날짜 startDate와 더할 기간 period를 구한다.
period만큼 월 수를 더하고, 여기서 예외처리가 중요하다. 12로 나눈 값만큼 연도에 더하고, 12로 나눈 나머지만큼의 값만 가진다.
그리고, 일자를 1씩 빼준다. 여기서, 2020년 1월 1일같은, 경계선에 대한 예외처리가 중요하다.
2번, 유효기간 날짜와 오늘 날짜를 비교하는 함수 compare함수를 만들었다. 오늘 날짜가 더 이후라면 expired를 반환하고, 아니라면 notExpired를 반환한다.
const datePlus = (startDate,period) => {
// 월 +1
let [year,month,date] = startDate.split('.').map(v=>+v);
month+= +period;
year += (parseInt(month/12));
month = month % 12;
date --;
if(date===0){
date=28;
month--;
}
if(month===0){
year--;
month=12;
}
return {year,month,date};
}
const compareDate = (expiration,today) => {
if(today.year>expiration.year) return 'expired';
if(today.year===expiration.year && today.month>expiration.month) return 'expired';
if(today.year===expiration.year && today.month===expiration.month && today.date>expiration.date) return 'expired';
return 'notExpired'
}
function solution(today, terms, privacies) {
var answer = [];
// 1. 유효기간 구하기
// 2. 유효기간 < today인지 구하기
const timesType={};
terms.forEach((term)=>{
term = term.split(' ')
timesType[term[0]] = term[1]
});
const [year,month,date] = today.split('.').map(v=>+v)
today = {year,month,date}
privacies = privacies.map((privacy,index)=>{
const [startDate,type] = privacy.split(' ');
const expiration = datePlus(startDate,timesType[type])
if (compareDate(expiration,today)==='expired'){
answer.push(index+1)
}
})
return answer;
}
🤔 2. 느낀 점
생각한대로 알고리즘을 짜고, 알고리즘대로 코드를 작성해내는 '구현' 문제이다. 그 알고리즘을 짜는 것이 상당히 중요하다는 것을 느꼈다. '유효기간을 구하고, 오늘 날짜와 비교하면 되겠지?' 생각에서 이를 단계로 만들어내는 능력. 그것이 알고리즘이구나.
그리고, 모든 테스트케이스를 통과하려면 각 조건들을 확인하여 예외처리를 잘 해야한다는 것을 느꼈다.
'코딩 테스트 > 프로그래머스' 카테고리의 다른 글
[프로그래머스] 수식 최대화 (0) | 2023.05.26 |
---|---|
[프로그래머스] 표 병합 (0) | 2023.05.21 |
[프로그래머스] 코딩테스트 공부 (0) | 2023.05.20 |
[프로그래머스] 표현 가능한 이진트리 (1) | 2023.05.19 |
[프로그래머스] 거리두기 확인하기 (0) | 2023.05.14 |
[프로그래머스] 방금 그곡 (1) | 2023.05.13 |
[프로그래머스] 후보키 (0) | 2023.05.12 |
[프로그래머스] 문자열 압축 (0) | 2023.05.11 |